Alert
Usage
Title
Use the title
prop to set the title of the Alert.
<template>
<UAlert title="Heads up!" />
</template>
Description
Use the description
prop to set the description of the Alert.
<template>
<UAlert title="Heads up!" description="You can change the primary color in your app config." />
</template>
Icon
Use the icon
prop to show an Icon.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
icon="i-lucide-terminal"
/>
</template>
Avatar
Use the avatar
prop to show an Avatar.
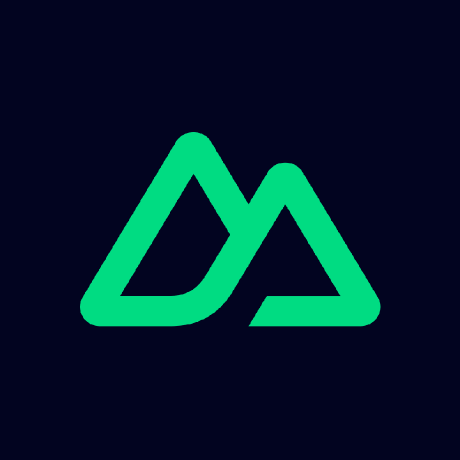
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
:avatar="{
src: 'https://github.com/nuxt.png'
}"
/>
</template>
Color
Use the color
prop to change the color of the Alert.
<template>
<UAlert
color="neutral"
title="Heads up!"
description="You can change the primary color in your app config."
icon="i-lucide-terminal"
/>
</template>
Variant
Use the variant
prop to change the variant of the Alert.
<template>
<UAlert
color="neutral"
variant="subtle"
title="Heads up!"
description="You can change the primary color in your app config."
icon="i-lucide-terminal"
/>
</template>
Close
Use the close
prop to display a Button to dismiss the Alert.
update:open
event will be emitted when the close button is clicked.<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
color="neutral"
variant="outline"
close
/>
</template>
You can pass any property from the Button component to customize it.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
color="neutral"
variant="outline"
:close="{
color: 'primary',
variant: 'outline',
class: 'rounded-full'
}"
/>
</template>
Close Icon
Use the close-icon
prop to customize the close button Icon. Defaults to i-lucide-x
.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
color="neutral"
variant="outline"
close
close-icon="i-lucide-arrow-right"
/>
</template>
Actions
Use the actions
prop to add some Button actions to the Alert.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
color="neutral"
variant="outline"
:actions="[
{
label: 'Action 1'
},
{
label: 'Action 2',
color: 'neutral',
variant: 'subtle'
}
]"
/>
</template>
Orientation
Use the orientation
prop to change the orientation of the Alert.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
color="neutral"
variant="outline"
orientation="horizontal"
:actions="[
{
label: 'Action 1'
},
{
label: 'Action 2',
color: 'neutral',
variant: 'subtle'
}
]"
/>
</template>
Examples
class
prop
Use the class
prop to override the base styles of the Alert.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
class="rounded-none"
/>
</template>
ui
prop
Use the ui
prop to override the slots styles of the Alert.
<template>
<UAlert
title="Heads up!"
description="You can change the primary color in your app config."
icon="i-lucide-rocket"
:ui="{
icon: 'size-11'
}"
/>
</template>
API
Props
Prop | Default | Type |
---|---|---|
as |
|
The element or component this component should render as. |
title |
| |
description |
| |
icon |
| |
avatar |
| |
color |
|
|
variant |
|
|
orientation |
|
|
actions |
Display a list of actions:
| |
close |
|
Display a close button to dismiss the alert.
|
closeIcon |
|
The icon displayed in the close button. |
ui |
|
Slots
Slot | Type |
---|---|
leading |
|
title |
|
description |
|
actions |
|
close |
|
Emits
Event | Type |
---|---|
update:open |
|
Theme
export default defineAppConfig({
ui: {
alert: {
slots: {
root: 'relative overflow-hidden w-full rounded-[calc(var(--ui-radius)*2)] p-4 flex gap-2.5',
wrapper: 'min-w-0 flex-1 flex flex-col',
title: 'text-sm font-medium',
description: 'text-sm opacity-90',
icon: 'shrink-0 size-5',
avatar: 'shrink-0',
avatarSize: '2xl',
actions: 'flex flex-wrap gap-1.5 shrink-0',
close: 'p-0'
},
variants: {
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
variant: {
solid: '',
outline: '',
soft: '',
subtle: ''
},
orientation: {
horizontal: {
root: 'items-center',
actions: 'items-center'
},
vertical: {
root: 'items-start',
actions: 'items-start mt-2.5'
}
},
title: {
true: {
description: 'mt-1'
}
}
},
compoundVariants: [
{
color: 'primary',
variant: 'solid',
class: {
root: 'bg-(--ui-primary) text-(--ui-bg)'
}
},
{
color: 'primary',
variant: 'outline',
class: {
root: 'text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
}
},
{
color: 'primary',
variant: 'soft',
class: {
root: 'bg-(--ui-primary)/10 text-(--ui-primary)'
}
},
{
color: 'primary',
variant: 'subtle',
class: {
root: 'bg-(--ui-primary)/10 text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
}
},
{
color: 'neutral',
variant: 'solid',
class: {
root: 'text-(--ui-bg) bg-(--ui-bg-inverted)'
}
},
{
color: 'neutral',
variant: 'outline',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg) ring ring-inset ring-(--ui-border)'
}
},
{
color: 'neutral',
variant: 'soft',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg-elevated)/50'
}
},
{
color: 'neutral',
variant: 'subtle',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg-elevated)/50 ring ring-inset ring-(--ui-border-accented)'
}
}
],
defaultVariants: {
color: 'primary',
variant: 'solid'
}
}
}
})
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import ui from '@nuxt/ui/vite'
export default defineConfig({
plugins: [
vue(),
ui({
ui: {
alert: {
slots: {
root: 'relative overflow-hidden w-full rounded-[calc(var(--ui-radius)*2)] p-4 flex gap-2.5',
wrapper: 'min-w-0 flex-1 flex flex-col',
title: 'text-sm font-medium',
description: 'text-sm opacity-90',
icon: 'shrink-0 size-5',
avatar: 'shrink-0',
avatarSize: '2xl',
actions: 'flex flex-wrap gap-1.5 shrink-0',
close: 'p-0'
},
variants: {
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
variant: {
solid: '',
outline: '',
soft: '',
subtle: ''
},
orientation: {
horizontal: {
root: 'items-center',
actions: 'items-center'
},
vertical: {
root: 'items-start',
actions: 'items-start mt-2.5'
}
},
title: {
true: {
description: 'mt-1'
}
}
},
compoundVariants: [
{
color: 'primary',
variant: 'solid',
class: {
root: 'bg-(--ui-primary) text-(--ui-bg)'
}
},
{
color: 'primary',
variant: 'outline',
class: {
root: 'text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
}
},
{
color: 'primary',
variant: 'soft',
class: {
root: 'bg-(--ui-primary)/10 text-(--ui-primary)'
}
},
{
color: 'primary',
variant: 'subtle',
class: {
root: 'bg-(--ui-primary)/10 text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
}
},
{
color: 'neutral',
variant: 'solid',
class: {
root: 'text-(--ui-bg) bg-(--ui-bg-inverted)'
}
},
{
color: 'neutral',
variant: 'outline',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg) ring ring-inset ring-(--ui-border)'
}
},
{
color: 'neutral',
variant: 'soft',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg-elevated)/50'
}
},
{
color: 'neutral',
variant: 'subtle',
class: {
root: 'text-(--ui-text-highlighted) bg-(--ui-bg-elevated)/50 ring ring-inset ring-(--ui-border-accented)'
}
}
],
defaultVariants: {
color: 'primary',
variant: 'solid'
}
}
}
})
]
})