PageHero
Usage
The PageHero component wraps your content in a Container while maintaining full-width flexibility making it easy to add background colors, images or patterns. It provides a flexible way to display content with an illustration in the default slot.
Ultimate Vue UI library
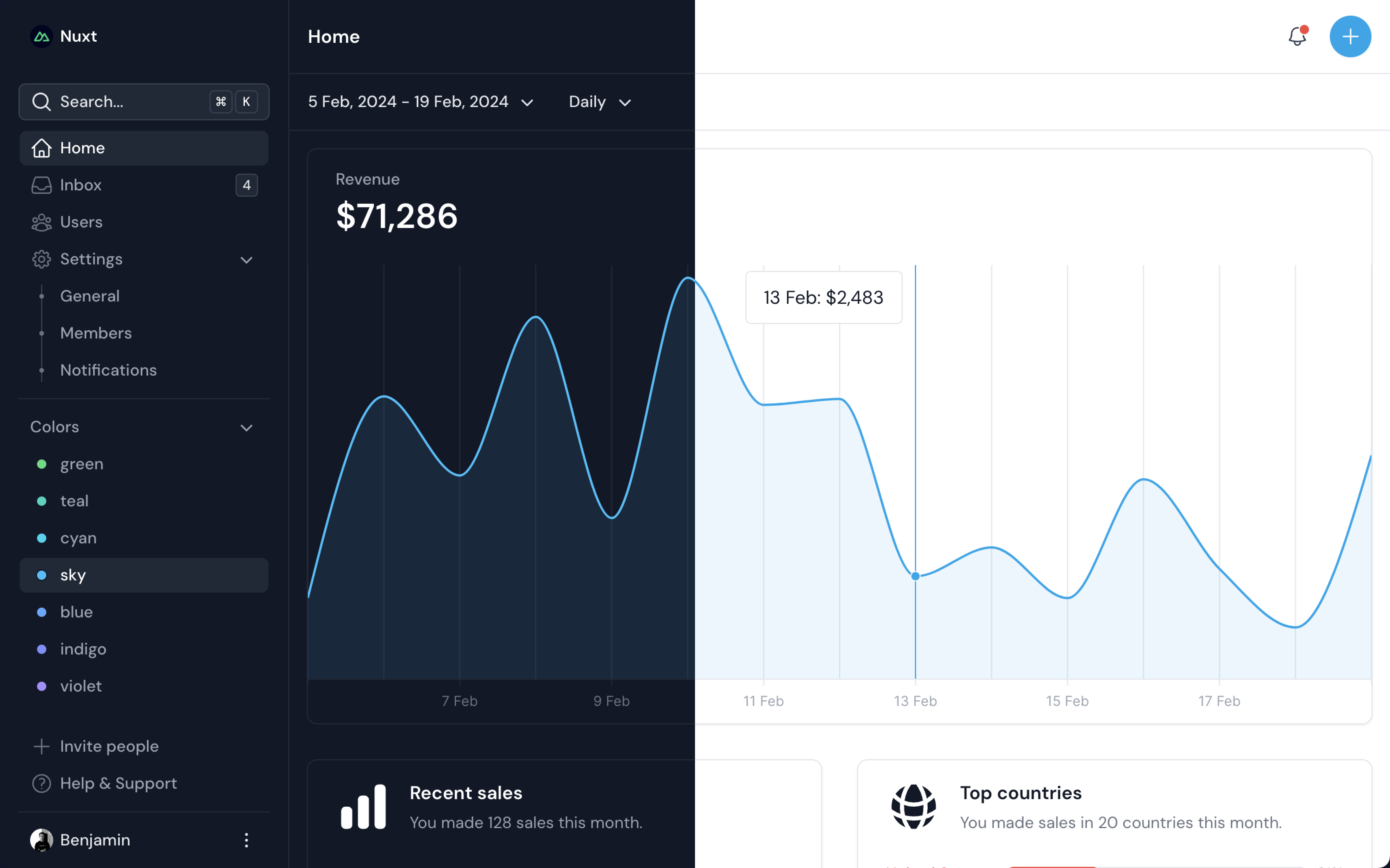
Title
Use the title
prop to set the title of the hero.
Ultimate Vue UI library
<template>
<UPageHero title="Ultimate Vue UI library" />
</template>
Description
Use the description
prop to set the description of the hero.
Ultimate Vue UI library
<template>
<UPageHero
title="Ultimate Vue UI library"
description="A Nuxt/Vue-integrated UI library providing a rich set of fully-styled, accessible and highly customizable components for building modern web applications."
/>
</template>
Headline
Use the headline
prop to set the headline of the hero.
Ultimate Vue UI library
<template>
<UPageHero
title="Ultimate Vue UI library"
description="A Nuxt/Vue-integrated UI library providing a rich set of fully-styled, accessible and highly customizable components for building modern web applications."
headline="New release"
/>
</template>
Links
Use the links
prop to display a list of Button under the description.
Ultimate Vue UI library
<script setup lang="ts">
const links = ref([
{
label: 'Get started',
to: '/getting-started',
icon: 'i-lucide-square-play'
},
{
label: 'Learn more',
to: '/getting-started/theme',
color: 'neutral',
variant: 'subtle',
trailingIcon: 'i-lucide-arrow-right'
}
])
</script>
<template>
<UPageHero
title="Ultimate Vue UI library"
description="A Nuxt/Vue-integrated UI library providing a rich set of fully-styled, accessible and highly customizable components for building modern web applications."
:links="links"
/>
</template>
Orientation
Use the orientation
prop to change the orientation with the default slot. Defaults to vertical
.
Ultimate Vue UI library
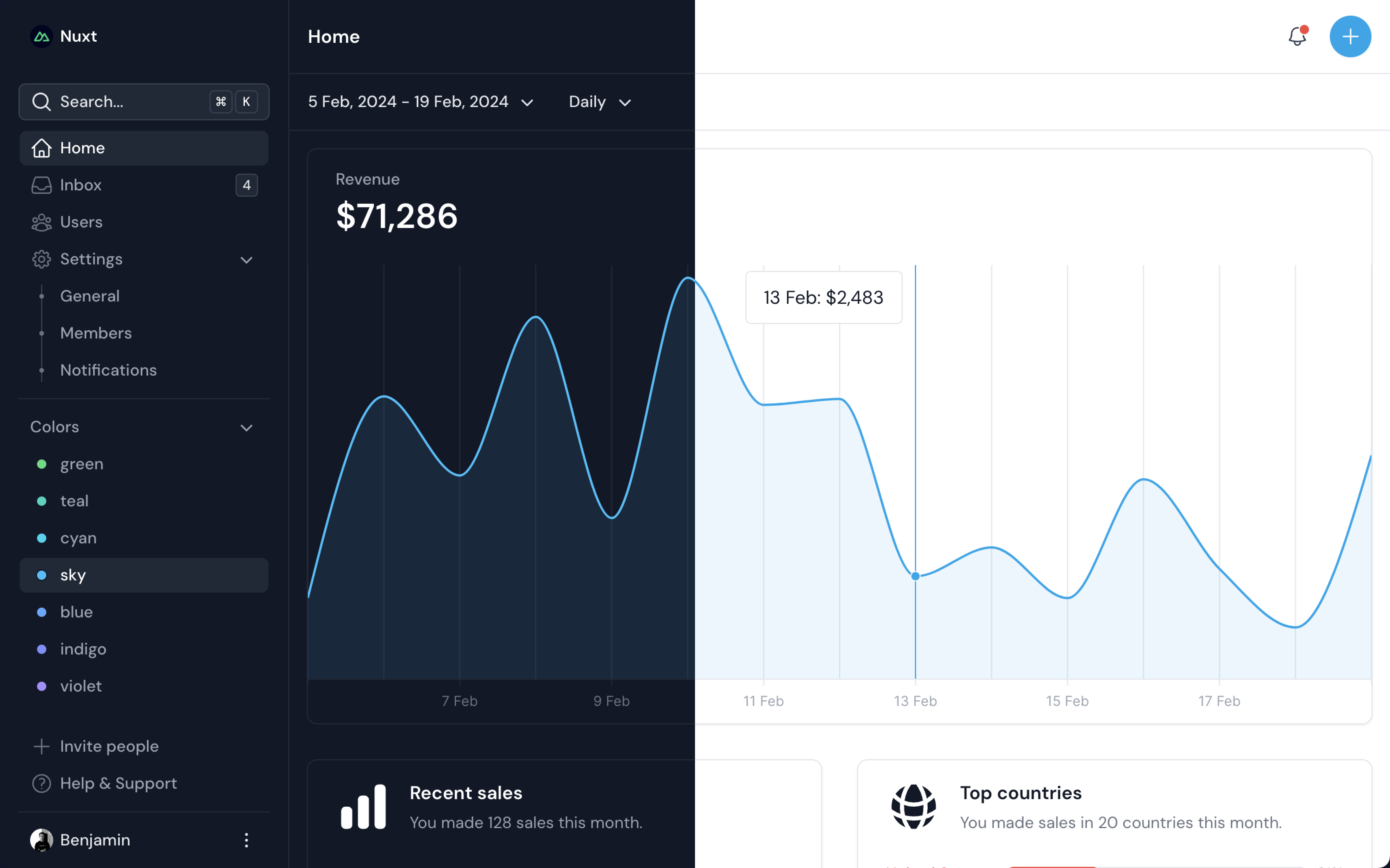
<script setup lang="ts">
const links = ref([
{
label: 'Get started',
to: '/getting-started',
icon: 'i-lucide-square-play'
},
{
label: 'Learn more',
to: '/getting-started/theme',
color: 'neutral',
variant: 'subtle',
trailingIcon: 'i-lucide-arrow-right'
}
])
</script>
<template>
<UPageHero
title="Ultimate Vue UI library"
description="A Nuxt/Vue-integrated UI library providing a rich set of fully-styled, accessible and highly customizable components for building modern web applications."
headline="New release"
orientation="horizontal"
:links="links"
>
<img
src="https://ui.nuxt.com/templates/dashboard1.png"
alt="App screenshot"
class="rounded-lg shadow-2xl ring ring-(--ui-border)"
/>
</UPageHero>
</template>
Reverse
Use the reverse
prop to reverse the orientation of the default slot.
Ultimate Vue UI library
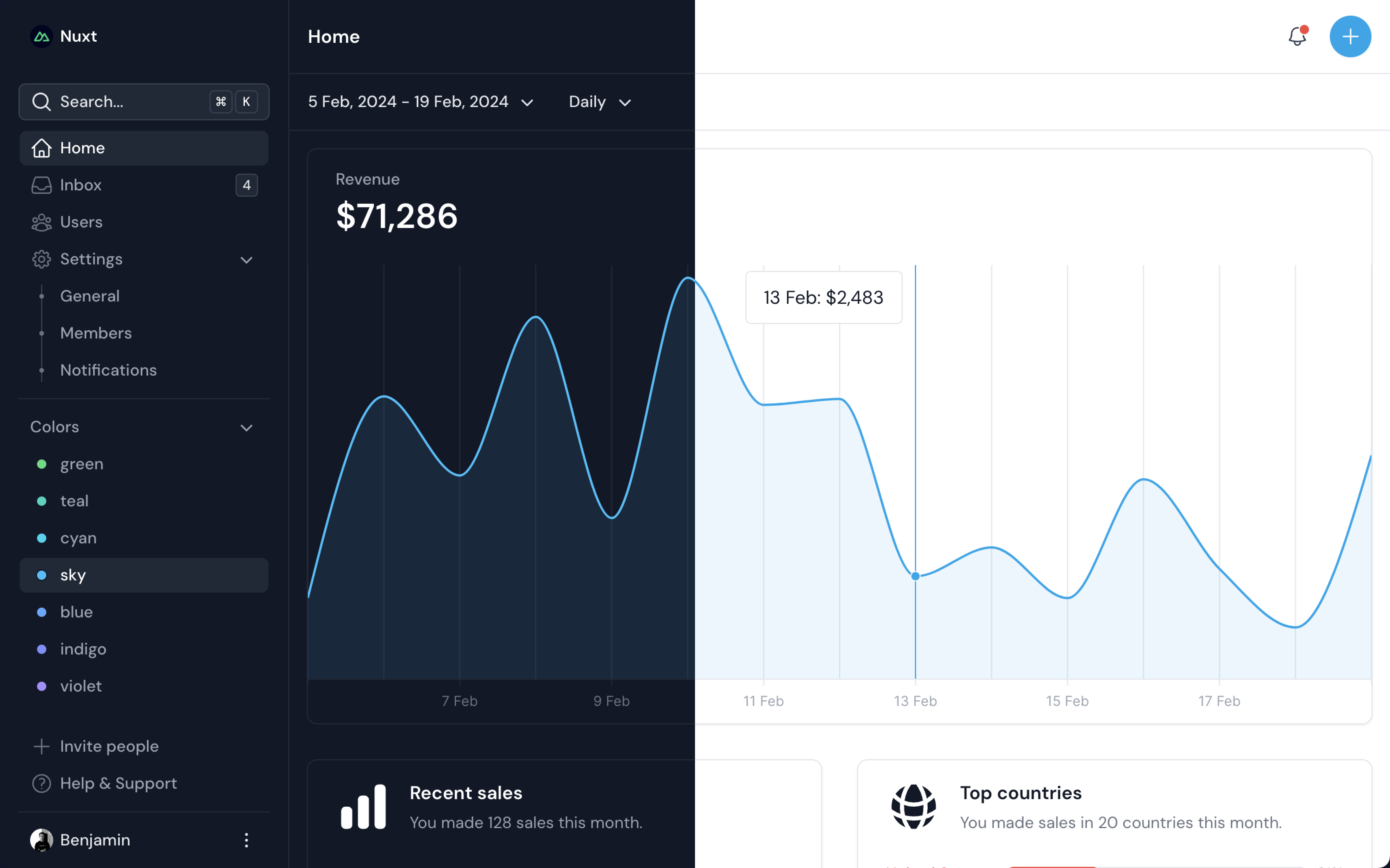
<script setup lang="ts">
const links = ref([
{
label: 'Get started',
to: '/getting-started',
icon: 'i-lucide-square-play'
},
{
label: 'Learn more',
to: '/getting-started/theme',
color: 'neutral',
variant: 'subtle',
trailingIcon: 'i-lucide-arrow-right'
}
])
</script>
<template>
<UPageHero
title="Ultimate Vue UI library"
description="A Nuxt/Vue-integrated UI library providing a rich set of fully-styled, accessible and highly customizable components for building modern web applications."
headline="New release"
orientation="horizontal"
reverse
:links="links"
>
<img
src="https://ui.nuxt.com/templates/dashboard1.png"
alt="App screenshot"
class="rounded-lg shadow-2xl ring ring-(--ui-border)"
/>
</UPageHero>
</template>
API
Props
Prop | Default | Type |
---|---|---|
as |
|
The element or component this component should render as. |
headline |
| |
title |
| |
description |
| |
links |
Display a list of Button under the description.
| |
orientation |
|
The orientation of the page hero. |
reverse |
|
Reverse the order of the default slot. |
ui |
|
Slots
Slot | Type |
---|---|
default |
|
top |
|
bottom |
|
headline |
|
title |
|
description |
|
links |
|
Theme
export default defineAppConfig({
uiPro: {
pageHero: {
slots: {
root: 'relative isolate',
container: 'flex flex-col lg:grid py-24 sm:py-32 lg:py-40 gap-16 sm:gap-y-24',
wrapper: '',
headline: 'mb-4',
title: 'text-5xl sm:text-7xl text-pretty tracking-tight font-bold text-(--ui-text-highlighted)',
description: 'text-lg sm:text-xl/8 text-(--ui-text-muted)',
links: 'mt-10 flex flex-wrap gap-x-6 gap-y-3'
},
variants: {
orientation: {
horizontal: {
container: 'lg:grid-cols-2 lg:items-center',
description: 'text-pretty'
},
vertical: {
container: '',
headline: 'justify-center',
wrapper: 'text-center',
description: 'text-balance',
links: 'justify-center'
}
},
reverse: {
true: {
wrapper: 'order-last'
}
},
headline: {
true: {
headline: 'font-semibold text-(--ui-primary) flex items-center gap-1.5'
}
},
title: {
true: {
description: 'mt-6'
}
}
}
}
}
})
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import ui from '@nuxt/ui/vite'
export default defineConfig({
plugins: [
vue(),
ui({
uiPro: {
pageHero: {
slots: {
root: 'relative isolate',
container: 'flex flex-col lg:grid py-24 sm:py-32 lg:py-40 gap-16 sm:gap-y-24',
wrapper: '',
headline: 'mb-4',
title: 'text-5xl sm:text-7xl text-pretty tracking-tight font-bold text-(--ui-text-highlighted)',
description: 'text-lg sm:text-xl/8 text-(--ui-text-muted)',
links: 'mt-10 flex flex-wrap gap-x-6 gap-y-3'
},
variants: {
orientation: {
horizontal: {
container: 'lg:grid-cols-2 lg:items-center',
description: 'text-pretty'
},
vertical: {
container: '',
headline: 'justify-center',
wrapper: 'text-center',
description: 'text-balance',
links: 'justify-center'
}
},
reverse: {
true: {
wrapper: 'order-last'
}
},
headline: {
true: {
headline: 'font-semibold text-(--ui-primary) flex items-center gap-1.5'
}
},
title: {
true: {
description: 'mt-6'
}
}
}
}
}
})
]
})