Badge
Usage
Label
Use the default slot to set the label of the Badge.
<template>
<UBadge>Badge</UBadge>
</template>
You can achieve the same result by using the label
prop.
<template>
<UBadge label="Badge" />
</template>
Color
Use the color
prop to change the color of the Badge.
<template>
<UBadge color="neutral">Badge</UBadge>
</template>
Variant
Use the variant
props to change the variant of the Badge.
<template>
<UBadge color="neutral" variant="outline">Badge</UBadge>
</template>
Size
Use the size
prop to change the size of the Badge.
<template>
<UBadge size="xl">Badge</UBadge>
</template>
Icon
Use the icon
prop to show an Icon inside the Badge.
<template>
<UBadge icon="i-lucide-rocket">Badge</UBadge>
</template>
Use the leading
and trailing
props to set the icon position or the leading-icon
and trailing-icon
props to set a different icon for each position.
<template>
<UBadge trailing-icon="i-lucide-arrow-right">Badge</UBadge>
</template>
Avatar
Use the avatar
prop to show an Avatar inside the Badge.
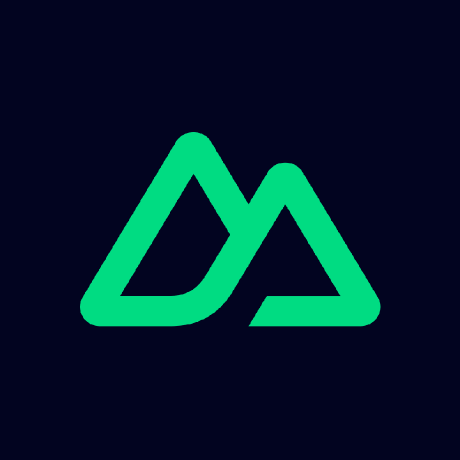
<template>
<UBadge
:avatar="{
src: 'https://github.com/nuxt.png'
}"
color="neutral"
variant="outline"
>
Badge
</UBadge>
</template>
Examples
class
prop
Use the class
prop to override the base styles of the Badge.
<template>
<UBadge class="font-bold rounded-full">Badge</UBadge>
</template>
API
Props
Prop | Default | Type |
---|---|---|
as |
|
The element or component this component should render as. |
label |
| |
color |
|
|
variant |
|
|
size |
|
|
trailingIcon |
Display an icon on the right side. | |
leadingIcon |
Display an icon on the left side. | |
leading |
When | |
trailing |
When | |
icon |
Display an icon based on the | |
avatar |
Display an avatar on the left side.
| |
ui |
|
Slots
Slot | Type |
---|---|
leading |
|
default |
|
trailing |
|
Theme
export default defineAppConfig({
ui: {
badge: {
slots: {
base: 'font-medium inline-flex items-center',
label: 'truncate',
leadingIcon: 'shrink-0',
leadingAvatar: 'shrink-0',
leadingAvatarSize: '',
trailingIcon: 'shrink-0'
},
variants: {
buttonGroup: {
horizontal: 'not-only:first:rounded-e-none not-only:last:rounded-s-none not-last:not-first:rounded-none',
vertical: 'not-only:first:rounded-b-none not-only:last:rounded-t-none not-last:not-first:rounded-none'
},
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
variant: {
solid: '',
outline: '',
soft: '',
subtle: ''
},
size: {
xs: {
base: 'text-[8px]/3 px-1 py-0.5 gap-1 rounded-[calc(var(--ui-radius))]',
leadingIcon: 'size-3',
leadingAvatarSize: '3xs',
trailingIcon: 'size-3'
},
sm: {
base: 'text-[10px]/3 px-1.5 py-1 gap-1 rounded-[calc(var(--ui-radius))]',
leadingIcon: 'size-3',
leadingAvatarSize: '3xs',
trailingIcon: 'size-3'
},
md: {
base: 'text-xs px-2 py-1 gap-1 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4'
},
lg: {
base: 'text-sm px-2 py-1 gap-1.5 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5'
},
xl: {
base: 'text-base px-2.5 py-1 gap-1.5 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-6',
leadingAvatarSize: '2xs',
trailingIcon: 'size-6'
}
}
},
compoundVariants: [
{
color: 'primary',
variant: 'solid',
class: 'bg-(--ui-primary) text-(--ui-bg)'
},
{
color: 'primary',
variant: 'outline',
class: 'text-(--ui-primary) ring ring-inset ring-(--ui-primary)/50'
},
{
color: 'primary',
variant: 'soft',
class: 'bg-(--ui-primary)/10 text-(--ui-primary)'
},
{
color: 'primary',
variant: 'subtle',
class: 'bg-(--ui-primary)/10 text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
},
{
color: 'neutral',
variant: 'solid',
class: 'text-(--ui-bg) bg-(--ui-bg-inverted)'
},
{
color: 'neutral',
variant: 'outline',
class: 'ring ring-inset ring-(--ui-border-accented) text-(--ui-text) bg-(--ui-bg)'
},
{
color: 'neutral',
variant: 'soft',
class: 'text-(--ui-text) bg-(--ui-bg-elevated)'
},
{
color: 'neutral',
variant: 'subtle',
class: 'ring ring-inset ring-(--ui-border-accented) text-(--ui-text) bg-(--ui-bg-elevated)'
}
],
defaultVariants: {
color: 'primary',
variant: 'solid',
size: 'md'
}
}
}
})
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import ui from '@nuxt/ui/vite'
export default defineConfig({
plugins: [
vue(),
ui({
ui: {
badge: {
slots: {
base: 'font-medium inline-flex items-center',
label: 'truncate',
leadingIcon: 'shrink-0',
leadingAvatar: 'shrink-0',
leadingAvatarSize: '',
trailingIcon: 'shrink-0'
},
variants: {
buttonGroup: {
horizontal: 'not-only:first:rounded-e-none not-only:last:rounded-s-none not-last:not-first:rounded-none',
vertical: 'not-only:first:rounded-b-none not-only:last:rounded-t-none not-last:not-first:rounded-none'
},
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
variant: {
solid: '',
outline: '',
soft: '',
subtle: ''
},
size: {
xs: {
base: 'text-[8px]/3 px-1 py-0.5 gap-1 rounded-[calc(var(--ui-radius))]',
leadingIcon: 'size-3',
leadingAvatarSize: '3xs',
trailingIcon: 'size-3'
},
sm: {
base: 'text-[10px]/3 px-1.5 py-1 gap-1 rounded-[calc(var(--ui-radius))]',
leadingIcon: 'size-3',
leadingAvatarSize: '3xs',
trailingIcon: 'size-3'
},
md: {
base: 'text-xs px-2 py-1 gap-1 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4'
},
lg: {
base: 'text-sm px-2 py-1 gap-1.5 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5'
},
xl: {
base: 'text-base px-2.5 py-1 gap-1.5 rounded-[calc(var(--ui-radius)*1.5)]',
leadingIcon: 'size-6',
leadingAvatarSize: '2xs',
trailingIcon: 'size-6'
}
}
},
compoundVariants: [
{
color: 'primary',
variant: 'solid',
class: 'bg-(--ui-primary) text-(--ui-bg)'
},
{
color: 'primary',
variant: 'outline',
class: 'text-(--ui-primary) ring ring-inset ring-(--ui-primary)/50'
},
{
color: 'primary',
variant: 'soft',
class: 'bg-(--ui-primary)/10 text-(--ui-primary)'
},
{
color: 'primary',
variant: 'subtle',
class: 'bg-(--ui-primary)/10 text-(--ui-primary) ring ring-inset ring-(--ui-primary)/25'
},
{
color: 'neutral',
variant: 'solid',
class: 'text-(--ui-bg) bg-(--ui-bg-inverted)'
},
{
color: 'neutral',
variant: 'outline',
class: 'ring ring-inset ring-(--ui-border-accented) text-(--ui-text) bg-(--ui-bg)'
},
{
color: 'neutral',
variant: 'soft',
class: 'text-(--ui-text) bg-(--ui-bg-elevated)'
},
{
color: 'neutral',
variant: 'subtle',
class: 'ring ring-inset ring-(--ui-border-accented) text-(--ui-text) bg-(--ui-bg-elevated)'
}
],
defaultVariants: {
color: 'primary',
variant: 'solid',
size: 'md'
}
}
}
})
]
})